Overview
JSONata is a query and tranformation language for JSON data. It allows you to declare the output using queries, operators and functions while formatting and parsing the data on the fly.
The official documentation does a great job of explaining the details of the language and providing a tool to test your JSONata expressions.
JSONata Expression Examples
To get a feel for what you can do with JSONata. Here are a couple of helpful examples to demonstrate how you can manipulate your data.
Simple Queries
We will start by looking at a few important queries and operations that can be applied to a typical Meraki API response.
Using the JSON from the API response, we can explore the data with some simple JSONata queries.
Description | JSONata | Result |
---|---|---|
Root of JSON | $ |
{object} |
List all values by property name | id |
["L_646829496481087792","L_646829496481091801"] |
Array Item | productTypes[0] |
"appliance" |
Array Items | productTypes[] |
["appliance","camera","switch","systemsManager","wireless"] |
Copy[
{
"id": "L_646829496481087792",
"organizationId": "537758",
"name": "London",
"productTypes": [
"appliance",
"camera",
"switch",
"systemsManager",
"wireless"
],
"timeZone": "Europe/London",
"tags": [
"Branch"
],
"enrollmentString": null,
"url": "https://n398.meraki.com/London-camera/n/gfcM6dvc/manage/usage/list",
"notes": ""
},
{
"id": "L_646829496481091801",
"organizationId": "537758",
"name": "Sydney",
"productTypes": [
"appliance",
"camera",
"systemsManager",
"wireless"
],
"timeZone": "Australia/Sydney",
"tags": [
"Retail"
],
"enrollmentString": null,
"url": "https://n398.meraki.com/Sydney-appliance/n/NyriCdvc/manage/usage/list",
"notes": "Sydney, Australia Retail Location"
}
]
^
Sorting
The ^
operation will sort the results based on a property name, where the thing to be sorted is within parenthesis. ^(sortMe)
In this example, the Device Statuses are being sorted on the status
value. Using the .
map operation, a new array of objects will be created. The serial
and status
fields are then defined to be used in the new JSON.
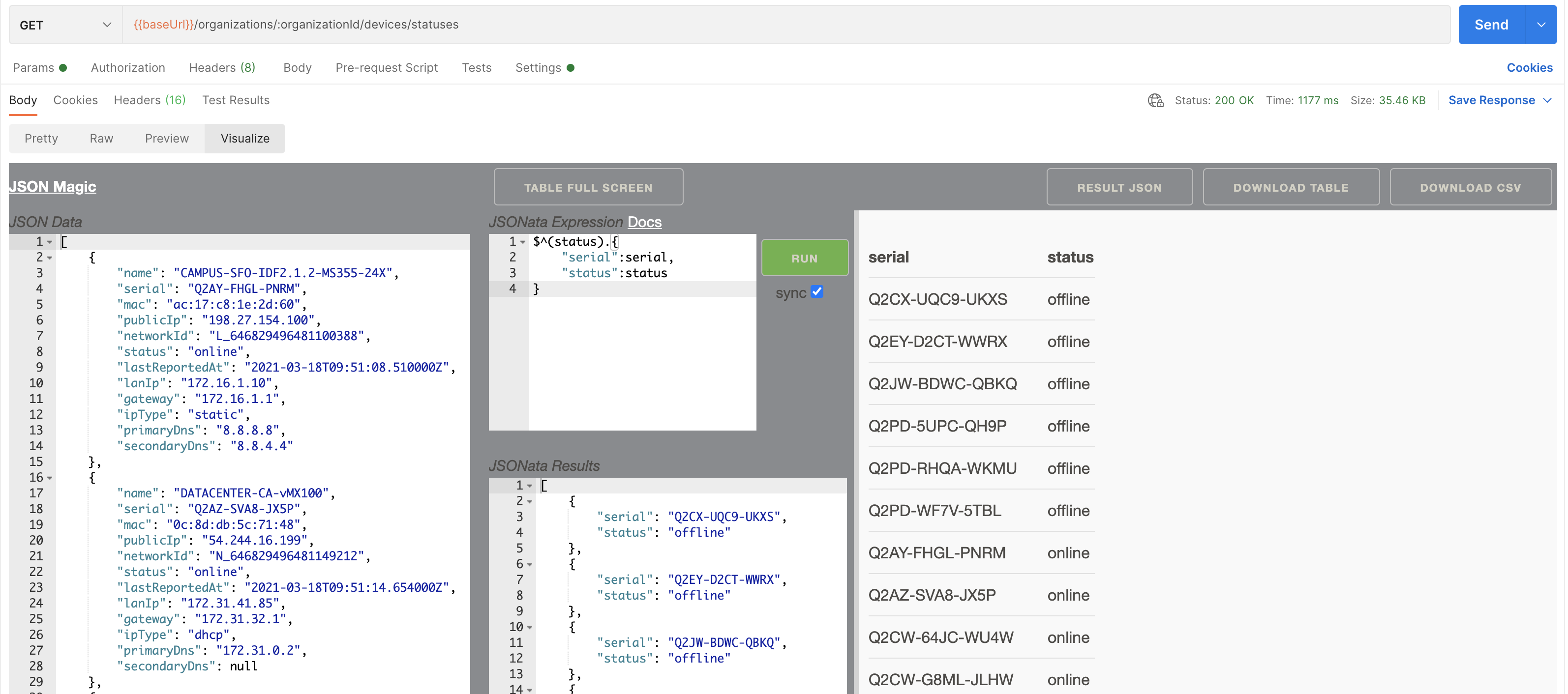
Copy$^(status).{
"serial":serial,
"status":status
}
Copy[
{
"name": "CAMPUS-SFO-IDF2.1.2-MS355-24X",
"serial": "Q2AY-FHGL-PNRM",
"mac": "ac:17:c8:1e:2d:60",
"publicIp": "198.27.154.100",
"networkId": "L_646829496481100388",
"status": "online",
"lastReportedAt": "2021-03-18T09:51:08.510000Z",
"lanIp": "172.16.1.10",
"gateway": "172.16.1.1",
"ipType": "static",
"primaryDns": "8.8.8.8",
"secondaryDns": "8.8.4.4"
},
{
"name": "DATACENTER-CA-vMX100",
"serial": "Q2AZ-SVA8-JX5P",
"mac": "0c:8d:db:5c:71:48",
"publicIp": "54.244.16.199",
"networkId": "N_646829496481149212",
"status": "online",
"lastReportedAt": "2021-03-18T09:51:14.654000Z",
"lanIp": "172.31.41.85",
"gateway": "172.31.32.1",
"ipType": "dhcp",
"primaryDns": "172.31.0.2",
"secondaryDns": null
},
{
"name": "CAMPUS-SFO-MDF1.1-MS425-16-CORE1",
"serial": "Q2CW-64JC-WU4W",
"mac": "88:15:44:a7:a3:9d",
"publicIp": "198.27.154.100",
"networkId": "L_646829496481100388",
"status": "online",
"lastReportedAt": "2021-03-18T09:51:18.983000Z",
"lanIp": "172.16.1.2",
"gateway": "172.16.1.1",
"ipType": "static",
"primaryDns": "8.8.8.8",
"secondaryDns": "8.8.4.4"
}
]
Copy[
{
"serial": "Q2AY-FHGL-PNRM",
"status": "online"
},
{
"serial": "Q2AZ-SVA8-JX5P",
"status": "online"
},
{
"serial": "Q2CW-64JC-WU4W",
"status": "online"
}
]
Update Values
Update properties and add custom markup.
In this example the data is being formatted to look nicer in a table.
The id
property is mapped to ID
and the productTypes
are now called Products
. The Network
property is an HTML hyperlink using the url
property for the href address and then displaying the name
.
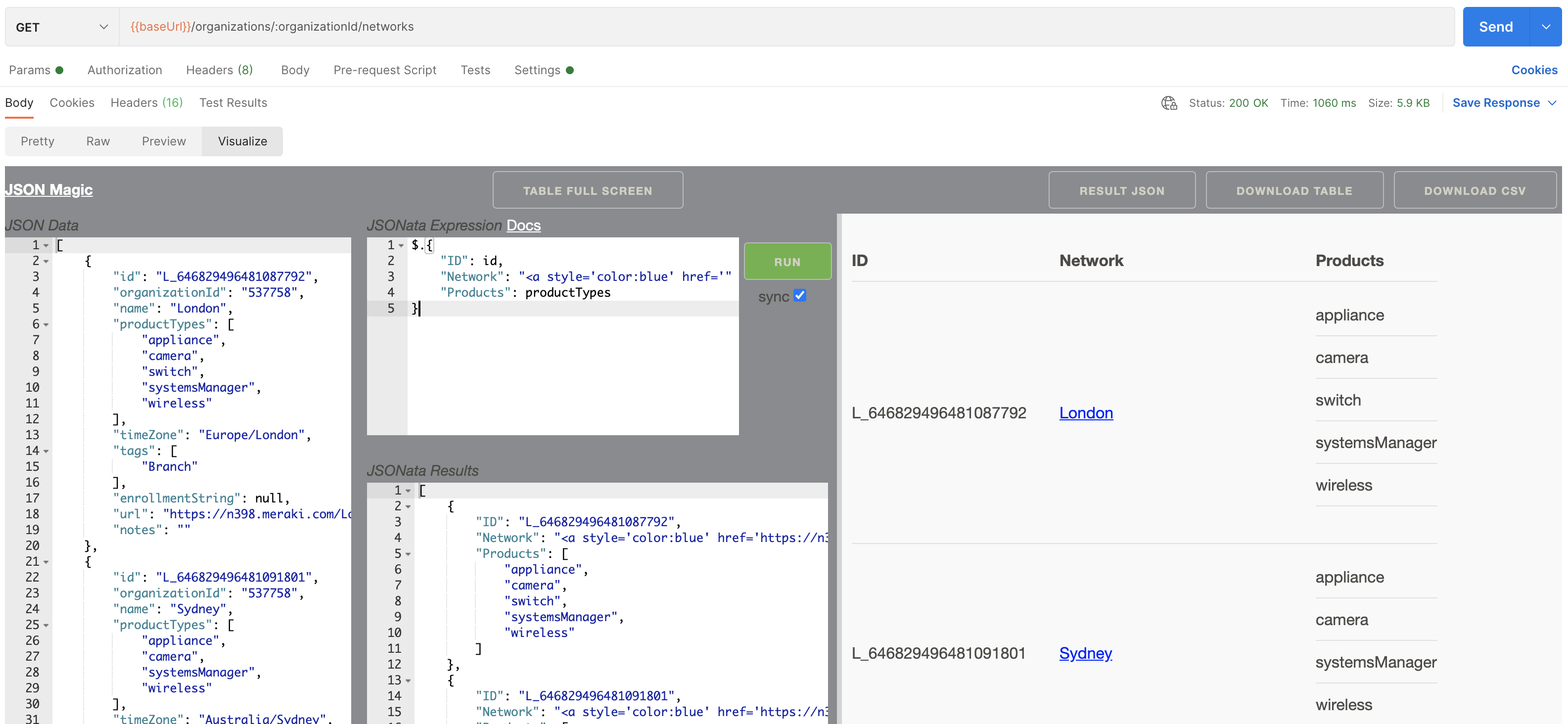
Copy$.{
"ID": id,
"Network": "<a style='color:blue' href='" & url & "'>" & name & "</a>",
"Products": productTypes
}
Copy[
{
"id": "L_646829496481087792",
"organizationId": "537758",
"name": "London",
"productTypes": [
"appliance",
"camera",
"switch",
"systemsManager",
"wireless"
],
"timeZone": "Europe/London",
"tags": [
"Branch"
],
"enrollmentString": null,
"url": "https://n398.meraki.com/London-camera/n/gfcM6dvc/manage/usage/list",
"notes": ""
},
{
"id": "L_646829496481091801",
"organizationId": "537758",
"name": "Sydney",
"productTypes": [
"appliance",
"camera",
"systemsManager",
"wireless"
],
"timeZone": "Australia/Sydney",
"tags": [
"Retail"
],
"enrollmentString": null,
"url": "https://n398.meraki.com/Sydney-appliance/n/NyriCdvc/manage/usage/list",
"notes": "Sydney, Australia Retail Location"
}
]
Copy[
{
"ID": "L_646829496481087792",
"Network": "<a style='color:blue' href='https://n398.meraki.com/London-camera/n/gfcM6dvc/manage/usage/list'>London</a>",
"Products": [
"appliance",
"camera",
"switch",
"systemsManager",
"wireless"
]
},
{
"ID": "L_646829496481091801",
"Network": "<a style='color:blue' href='https://n398.meraki.com/Sydney-appliance/n/NyriCdvc/manage/usage/list'>Sydney</a>",
"Products": [
"appliance",
"camera",
"systemsManager",
"wireless"
]
}
]
Filtering Data
Filter properties by value.
In this example we list only clients that have the manfuacturer name of "Cisco Meraki"
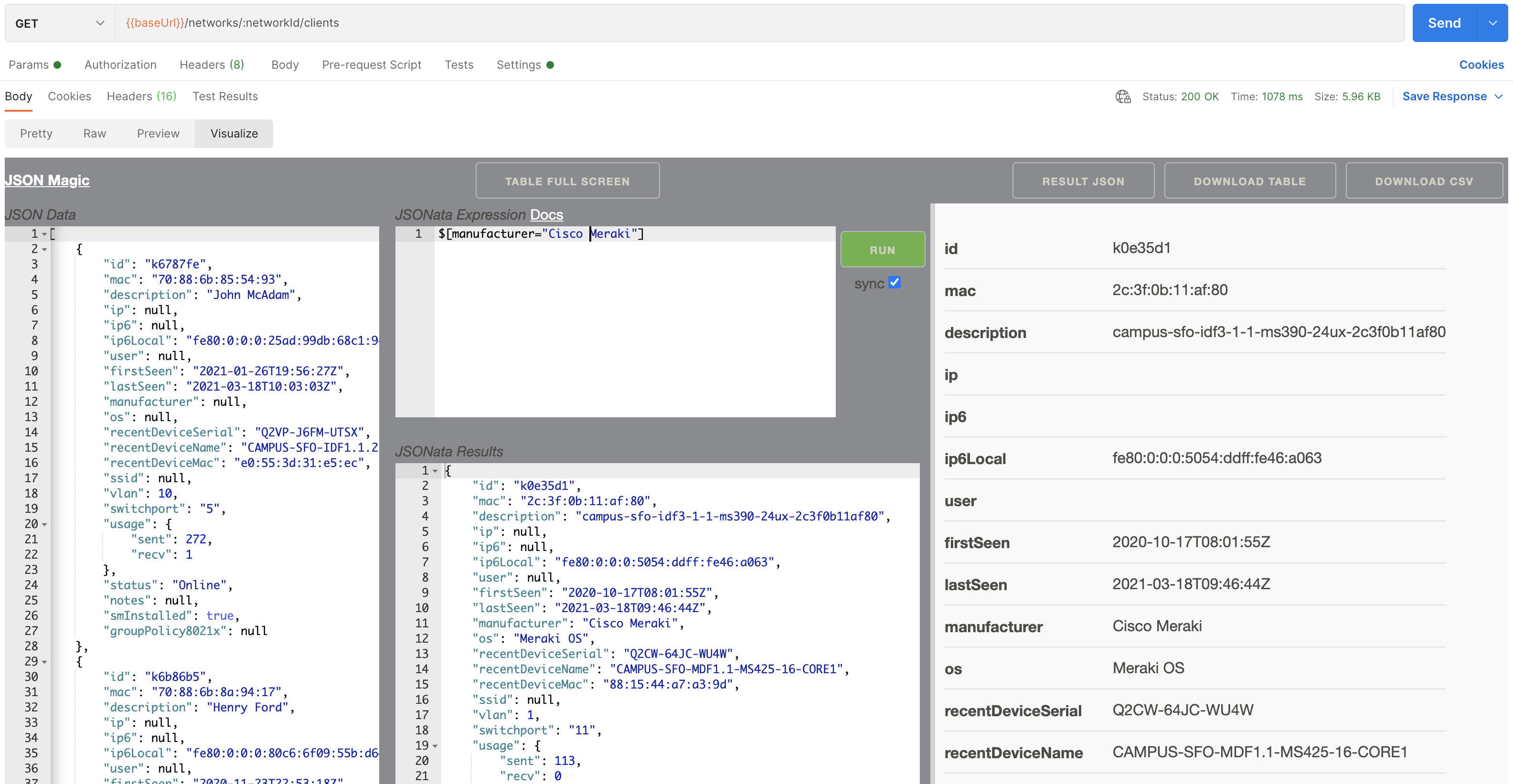
Copy$[manufacturer="Cisco Meraki"]
Copy[
{
"id": "k6787fe",
"mac": "70:88:6b:85:ff:ff",
"description": "John Miles",
"ip": null,
"ip6": null,
"ip6Local": "fe80:0:0:0:25ad:99db:68c1:ffff",
"user": null,
"firstSeen": "2021-01-26T19:56:27Z",
"lastSeen": "2021-03-18T10:03:03Z",
"manufacturer": null,
"os": null,
"recentDeviceSerial": "Q2VP-J6FM-XXXX",
"recentDeviceName": "CAMPUS-SFO-IDF1.1.2-MS350-24",
"recentDeviceMac": "e0:55:3d:31:ff:ff",
"ssid": null,
"vlan": 10,
"switchport": "5",
"usage": {
"sent": 272,
"recv": 1
},
"status": "Online",
"notes": null,
"smInstalled": true,
"groupPolicy8021x": null
},
{
"id": "k0e35d1",
"mac": "2c:3f:0b:11:af:80",
"description": "campus-sfo-idf3-1-1-ms390-24ux-2c3f0b11af80",
"ip": null,
"ip6": null,
"ip6Local": "fe80:0:0:0:5054:ddff:fe46:ffaa",
"user": null,
"firstSeen": "2020-10-17T08:01:55Z",
"lastSeen": "2021-03-18T09:46:44Z",
"manufacturer": "Cisco Meraki",
"os": "Meraki OS",
"recentDeviceSerial": "Q2CW-64JC-FFFF",
"recentDeviceName": "CAMPUS-SFO-MDF1.1-MS425-16-CORE1",
"recentDeviceMac": "88:15:44:a7:a3:9d",
"ssid": null,
"vlan": 1,
"switchport": "11",
"usage": {
"sent": 113,
"recv": 0
},
"status": "Offline",
"notes": null,
"smInstalled": false,
"groupPolicy8021x": null
}
]
Copy{
"id": "k0e35d1",
"mac": "2c:3f:0b:11:af:80",
"description": "campus-sfo-idf3-1-1-ms390-24ux-2c3f0b11af80",
"ip": null,
"ip6": null,
"ip6Local": "fe80:0:0:0:5054:ddff:fe46:ffaa",
"user": null,
"firstSeen": "2020-10-17T08:01:55Z",
"lastSeen": "2021-03-18T09:46:44Z",
"manufacturer": "Cisco Meraki",
"os": "Meraki OS",
"recentDeviceSerial": "Q2CW-64JC-FFFF",
"recentDeviceName": "CAMPUS-SFO-MDF1.1-MS425-16-CORE1",
"recentDeviceMac": "88:15:44:a7:a3:9d",
"ssid": null,
"vlan": 1,
"switchport": "11",
"usage": {
"sent": 113,
"recv": 0
},
"status": "Offline",
"notes": null,
"smInstalled": false,
"groupPolicy8021x": null
}