Automation API Docs
The Automation
API allows developers to interact with the backend of the low-to-no-code Automation engine in Cisco XDR. You can leverage this to manage objects, retrieve Workflow Run information and even execute Workflows via API. If you want to learn how to create Workflows, we recommend the Learning Labs that are linked in this document, or checking out the Workflow Best Practices. To learn how to publish to the XDR Automate Exchannge, please also review those instructions.
Note: The Automation API has a different source than the other Cisco XDR APIs, resulting in slight differences. For example, the Automation API is versioned, whereas the other API endpoints are usually not.
Required API Scope: for the Automation API you will need the
ao
API scope. You can also create an Automation Workflow, and leverage the built-in Cisco XDR Automation TargetAutomation API
, as described in the Getting Started. The interactive API Docs also allows you to test (read-only) out the Automation API with the pre-filled credentials.
Use Cases
- Create or update HTTP targets to make API calls.
- Query available targets while running a workflow.
- Query approval tasks.
- Run a workflow via API trigger.
How to use the API Docs
Use the interactive documentation to explore the Automation
API endpoints. Each request will have a complete description of all the required parameters and it also allows you to instantly try it out in the online console. Code templates are also provided for you to quickly build scripts.
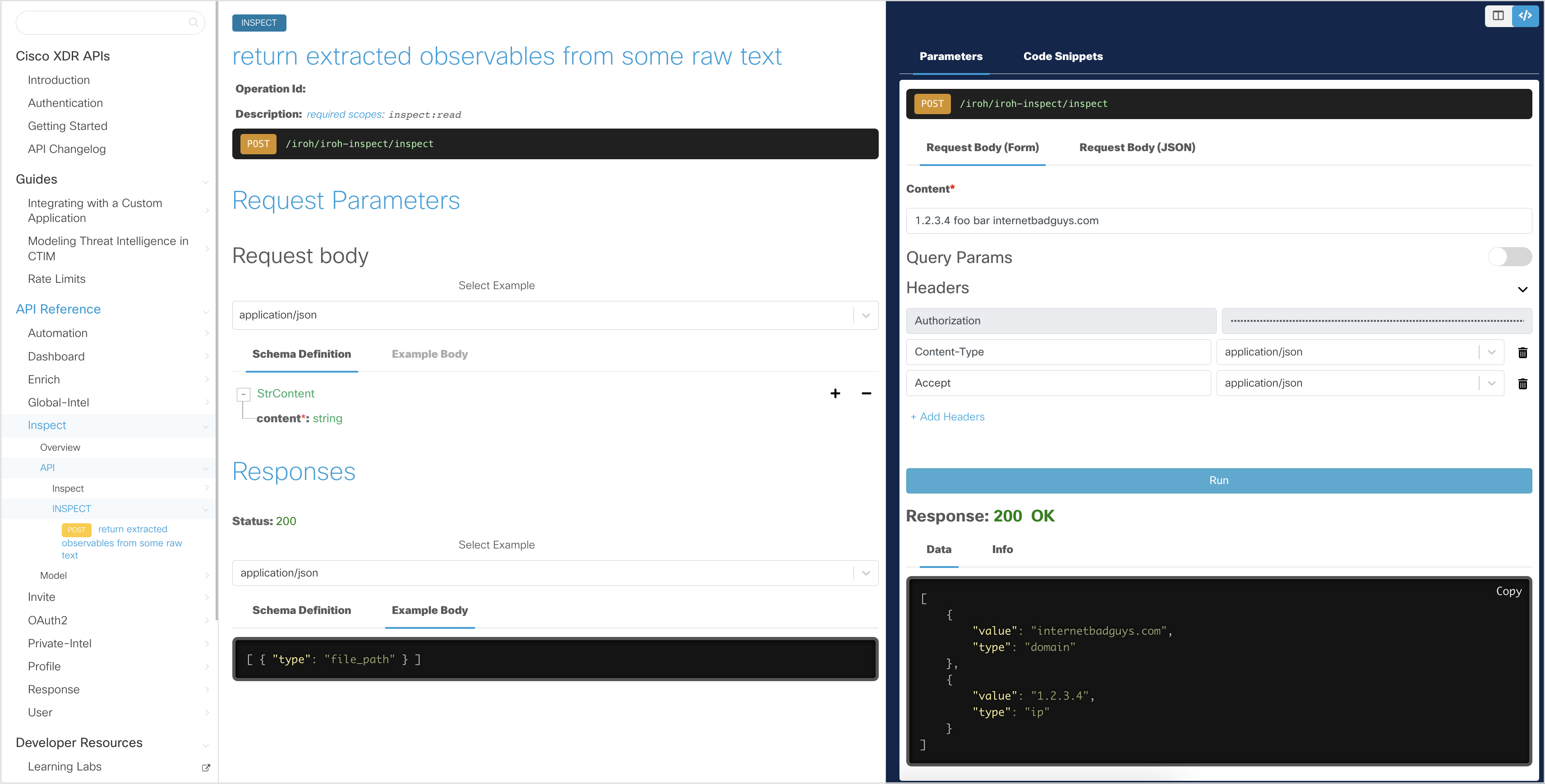
In the interactive explorer, the Client ID
and Client Secret
has been pre-filled and will allow you to make read-only API requests. These credentials will allow you to get an Access Token
, which will be stored for subsequent API requests and regenerated when it expires.
Note: The interactive documentation uses read-only credentials and the try it out feature will only work with
GET
and selectedPOST
requests. To do other type of requests you can create your own API credentials and use the method of choice to make them (e.g. cURL, Postman, Python, Automation Workflow)
Generate an Access Token
In the interactive API explorer, the Access Token
is automatically generated using the pre-filled Client ID
and Client Secret
so you do not need to generate it yourself.
If you want to understand how the Access Token
is generated from the Client ID
and Client Secret
credentials, take a look at the Authentication page.
For detailed instructions on how to use the interactive API documentation (or your own Python script), see the Getting Started page.
Download the Automation OpenAPI Specification
Download the Automation OpenAPI specification (OAS) file here.
Sample Code
Below is an example of how to use the Automation
API.
import json
import requests
# create headers for API request (See the OAuth2 overview page for sample code to generate an access token)
access_token = 'eyJhbGciO....bPito5n5Q' # truncated example, generate JWT token separately
bearer_token = 'Bearer ' + access_token
# get a list of all the workflows
url = 'https://automate.us.security.cisco.com/api/v1/workflows'
headers = {
'Authorization': bearer_token,
'Content-Type':'application/json',
'Accept':'application/json'
}
response = requests.get(url, headers=headers)
print(response.text)
if response.status_code == 200:
# convert the response to a dict object
response_json = json.loads(response.text)
# iterate through the workflows
for workflow in response_json:
# get the values from the workflow (remainder values are accessed in the same way)
id = workflow['id']
name = workflow['name']
type = workflow['type']