Popover Service
Class finesse.containerservices.PopoverService
Cisco Finesse voice component and gadgets hosting digital services uses the finesse.containerservices.PopoverService to display a popover for incoming calls and chat events.
Note | The popover is different from Cisco Finesse toaster notification. Toaster is a built-in browser notification, and it appears only if the agent desktop's browser tab is in the background. |
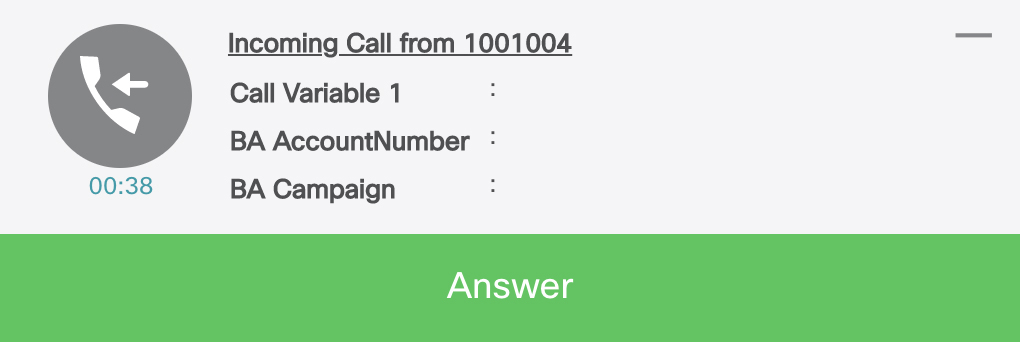
Object Definitions
The finesse.containerservices.PopoverService class uses specific data objects as inputs. The properties and their values of the object are JSON schema compliant. The format is defined below.
actionData
The actionData object defines the set of actions that can be taken and the buttons to be displayed popover.
Sample actionData Object
The payload details are explained in the table below.
Key |
Type |
Description |
---|---|---|
dismissible |
Boolean |
Determines whether the popover must be manually dismissed.
|
keepMaximised |
Boolean |
Determines whether the popover must be maximized or minimized.
|
clientIdentifier |
String |
Unique identifier across all popovers. Used in the callback for popover events. |
requiredActionText (optional) |
String |
The text in the popover that describes the user action. |
buttons (optional) |
Array |
Options for the action buttons (maximum two) in the popover. |
-->id |
String |
Unique identifier across all popovers. Used in the callback for popover events. |
-->label |
String |
The text of the action button. |
-->type |
Enum |
The color of the button.
|
-->hoverText (optional) |
String |
The tooltip when you hover the mouse pointer over the 'requiredActionText' (popover) when the text is truncated. |
-->confirmButtons (optional) |
Object |
The confirmation message with the buttons in response to the user action. |
–-->id |
String |
Unique identifier of the confirmation button. |
–-->label |
String |
The label on the button. |
–-->hoverText |
String |
The tooltip message on the confirmation button. |
The following method can be used to get the schema for the actionData object.
bannerData
The bannerData object helps to configure the data displayed on the popover.
Sample bannerData Object
The payload details are explained in the table below.
Key |
Type |
Description |
---|---|---|
icon |
Object |
The icon displayed in the popover. |
-->type |
Enum |
The type of icon in the popover. For more information, see Cisco Common Desktop Stock Icon Names with Image. |
–>value |
String |
The display name of the icon. |
content (optional) |
Array |
The list of six names or value pairs to be displayed in the popover. |
-->name |
String |
The display name of an individual name or value pair to be displayed on the popover. |
-->value |
String |
The corresponding value of the name for the individual name or value pair to be displayed on the popover. |
headerContent |
Object |
The title of the popover when it is maximized or minimized. |
-->maxHeaderTitle |
String |
The title of the popover when it is maximized. |
-->minHeaderTitle |
String |
The title of the popover when it is minimized. |
The following method can be used to get the schema for the bannerData object.
timerData
The timerData object helps configure the timer that displayed on the popover, which indicates the time left for the popover to be dismissed.
Sample timerData Object
The payload details are explained in the table below.
Key |
Type |
Description |
---|---|---|
displayTimeoutInSecs (mandatory) |
Integer |
The popover timeout in seconds. The minimum is 3 seconds and the maximum is 3600 seconds. –1 refers to no upper limit. |
display |
Boolean |
Determines whether the timer must be displayed on the popover.
|
counterType |
Enum |
Determines the direction in which time in the popover should be updated.
|
The following method can be used to get the schema for the timerData object.
Methods
dismissPopover(popoverId)
Dismisses the popover with the given popover Id.
Parameters
Name |
Type |
Description |
Required |
---|---|---|---|
popoverId |
String |
Unique identifier of the popover to be dismissed. This Id is returned from the showPopover call. |
Yes |
Throws
{Error}
If the service is not initialized.
generatePayload(isExistingPopover, popoverId, bannerData, timerData, actionData)
Generates a single payload for use by the popover service.
Parameters
Name |
Type |
Description |
Required |
---|---|---|---|
isExistingPopover |
Boolean |
Determines whether the popover is shown in the Finesse desktop.
|
Yes |
popoverId |
String |
Unique identifier of the popover to be generated. This Id is returned from the showPopover call. |
Yes |
bannerData |
Object |
The data displayed on the popover. For example, Customer Information such as name and phone number. For more information on the payload and description, see bannerData. |
Yes |
timerData |
Object |
The time left for the popover to be dismissed. The duration is displayed in seconds. For example, 00:38. For more information on the payload and description, see timerData. |
Yes |
actionData |
Object |
Describes the set of actions to be taken on the displayed popover. For example, Answer and Decline. For more information on the payload and description, see actionData. |
Yes |
Throws
{Error}
If the popoverData is not as per defined format.
init(ContainerService)
Initiates the PopoverService which is used by gadgets.
Parameters
Name |
Type |
Description |
Required |
---|---|---|---|
ContainerService |
Function |
Provides container level services for gadget developers. Gadgets can utilize the container dialogs and event handling to add or remove a service. |
Yes |
Returns
{finesse.containerservices.PopoverService}
The initiated finesse.containerServices.PopoverService reference.
showPopover(bannerData, timerData, actionData, actionHandler)
Shows a popover with the specified data. The user interaction or timeout of the popover is notified to the gadget through the registered actionHandler.
Note | When there is a new popover, the older popover is minimized except the popover related to voice calls. |
Parameters
Name |
Type |
Description |
Required | |
---|---|---|---|---|
bannerData |
Object |
The data displayed on the popover. For example, Customer Information such as name and phone number. For more information on the payload and description, see bannerData. |
Yes | |
timerData |
Object |
The time left for the popover to be dismissed. The duration is displayed in seconds. For example, 00:38. For more information on the payload and description, see timerData. |
Yes | |
actionData |
Object |
Describes the set of actions to be taken on the displayed popover. For example, Answer and Decline. For more information on the payload and description, see actionData. |
Yes | |
actionHandler |
Function |
Handler function that gets invoked for the events that are associated with the user interactions. |
Yes | |
-->popoverId |
String |
Unique identifier of the popover to be generated. This Id is returned from the showPopover call. |
Yes | |
-->source |
String |
Unique identifier of the source which generates the event. For example, 'btn_[id]_click', 'dismissed', or 'timeout'. |
Yes |
Throws
{Error}
If the popoverData is not as per defined format.
Returns
{String}
The popover Id and can be used for subsequent interaction with the service.
updatePopover(popoverId, bannerData, timerData, actionData, actionHandler)
Updates an active popover's displayed content.
Parameters
Name |
Type |
Description |
Required | |
---|---|---|---|---|
popoverId |
String |
Unique identifier of the popover to be generated. This Id is returned from the showPopover call. |
Yes | |
bannerData |
Object |
The data displayed on the popover. For example, Customer Information such as name and phone number. For more information on the payload and description, see bannerData. |
Yes | |
timerData |
Object |
The time left for the popover to be dismissed. The duration is displayed in seconds. For example, 00:38. For more information on the payload and description, see timerData. |
Yes | |
actionData |
Object |
Describes the set of actions to be taken on the displayed popover. For example, Answer and Decline. For more information on the payload and description, see actionData. |
Yes | |
actionHandler |
Function |
Handler function that gets invoked for the events that are associated with the user interactions. |
Yes |
Throws
{Error}
If the popoverData is not as per defined format.